How to use Intl DateTimeFormat with TypeScript?
When I first started using Intel.dateTimeFormatter, I ran into a problem that wasn't obvious, at least to me. But the problem turned out to be that I needed to explicitly specify the data type in the object options. And in this article, I will tell you how to solve this Intl.DateTimeFormat TypeScript error problem.
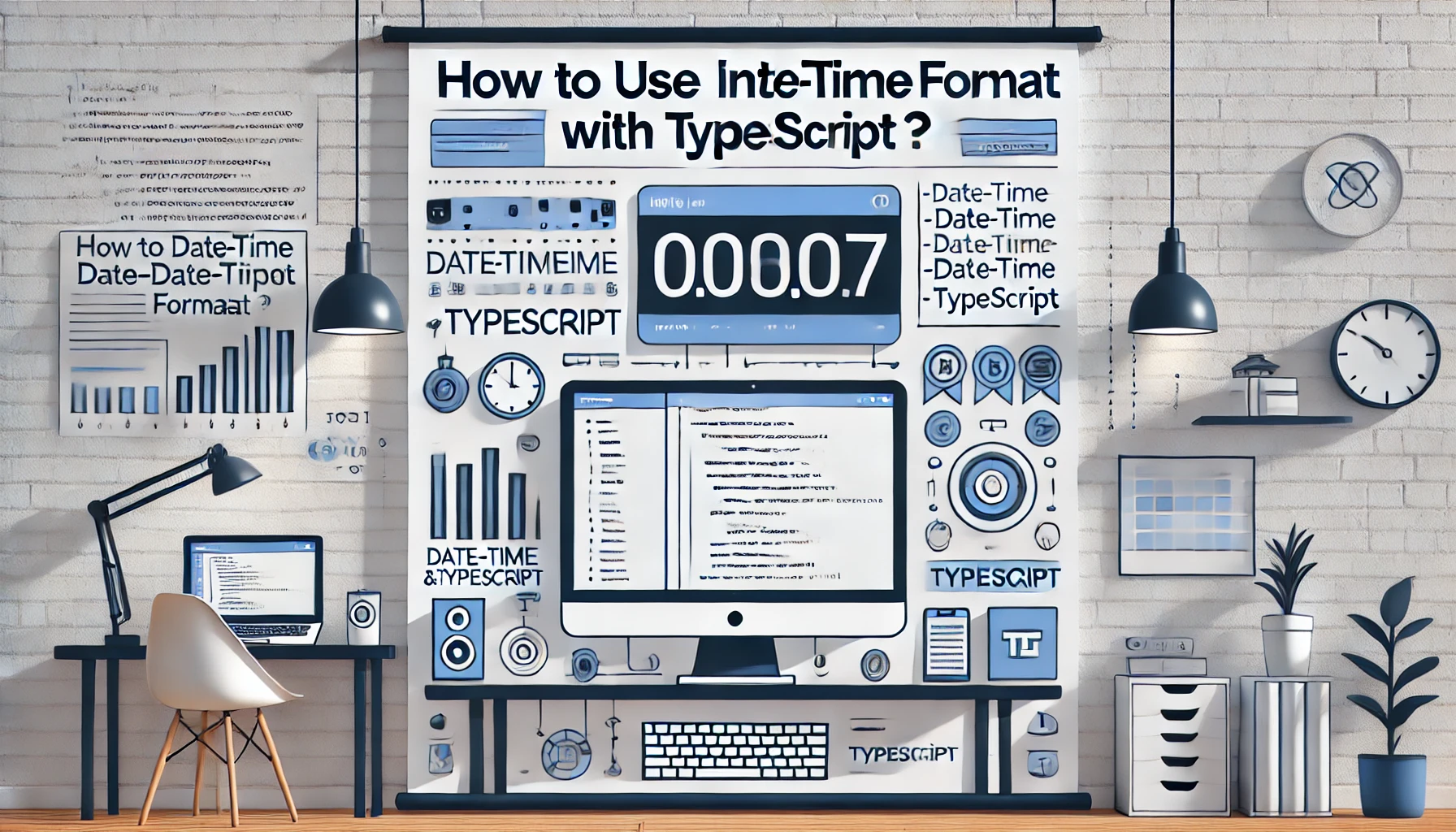
What is the Problem?
The problem looks like this. When you start using an object with options and add it to Intl.DateTimeFormat, you get the following error:
No overload matches this call.
Overload 1 of 2, '(locales?: LocalesArgument, options?: DateTimeFormatOptions): DateTimeFormat', gave the following
error.
Overload 2 of 2, '(locales?: string | string[], options?: DateTimeFormatOptions): DateTimeFormat', gave the following
error.ts(2769)
const fullOptions: {
year: string;
month: string;
day: string;
hour: string;
minute: string;
timeZone: string;
hour12: boolean;
}
const fullOptions = {
year: 'numeric',
month: 'short',
day: '2-digit',
hour: '2-digit',
minute: '2-digit',
timeZone: 'CET',
hour12: false,
};
formattedDate = new Intl.DateTimeFormat('en-GB', fullOptions).format(d);
How to Fix the Error
This error appeared for a rather simple reason. When we use an object with literal types, we must specify exactly which types will be there. To do this, we need to go into Intl and get the object with options from there, and assign this type to our object. Below, I provide a working example of the code.
const fullOptions: Intl.DateTimeFormatOptions = {
year: 'numeric',
month: 'short',
day: '2-digit',
hour: '2-digit',
minute: '2-digit',
timeZone: 'CET',
hour12: false,
};
formattedDate = new Intl.DateTimeFormat('en-GB', fullOptions).format(d);
But let me still try to explain to you why this is happening.
In TypeScript, if we create a variable with text, the type of this variable will not be string but only the text itself from the variable.
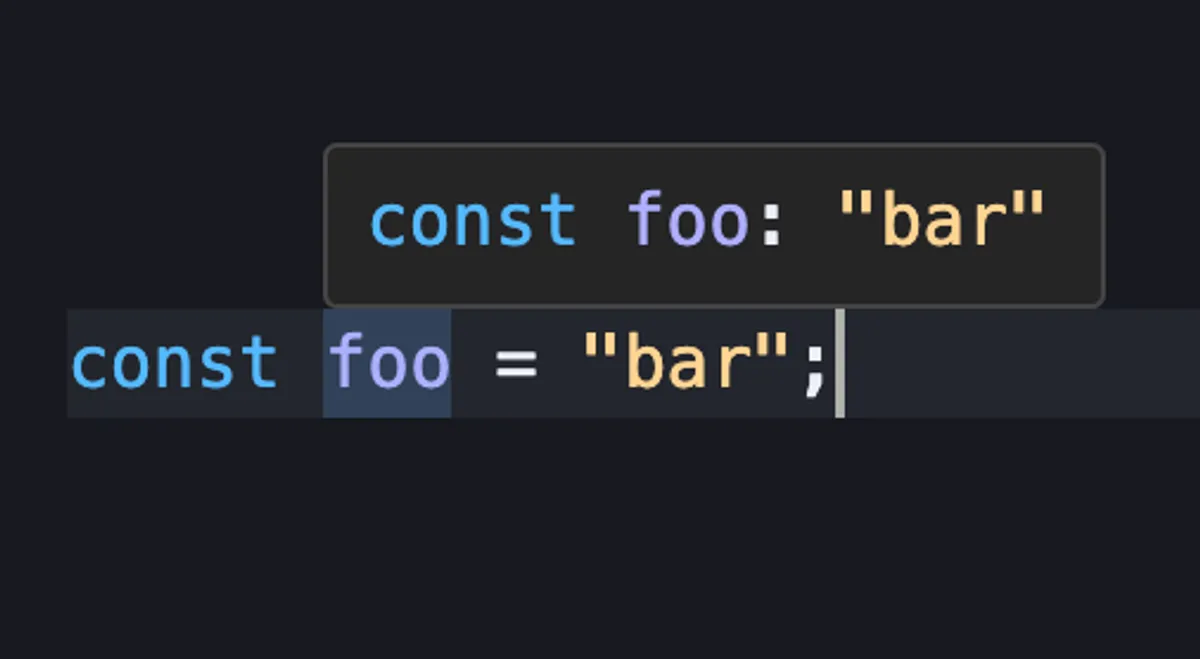
Similarly, if we use a literal type, the variable will not be a string type but only one of the literals.
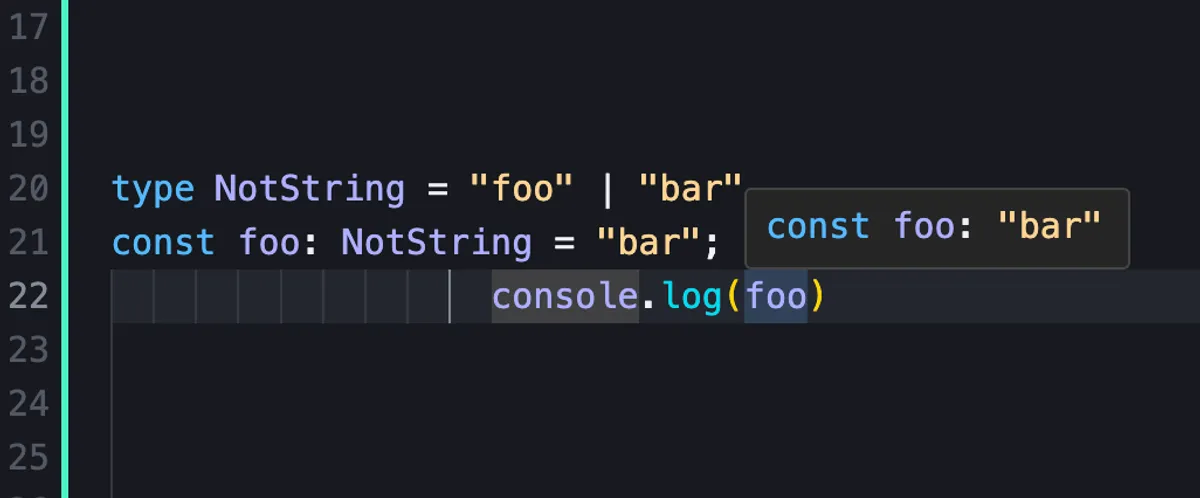
Thus, we need to be more careful with our types and remember that not everything that seems like a string is actually a string.