Essential Git Commands for Everyday Use and Rare but Important Operations
In this article, I will describe the Git commands that I use daily, as well as those that are used less frequently but are essential for handling complex scenarios in Git. The focus will be on fundamental commands such as git status, git add, git commit, and git push, which form the backbone of everyday version control operations. Additionally, I will share commands that are not used as often but play a crucial role in project management and solving advanced tasks. This article will be periodically updated with new commands as I encounter and learn them, serving as a handy reference for efficient Git usage.
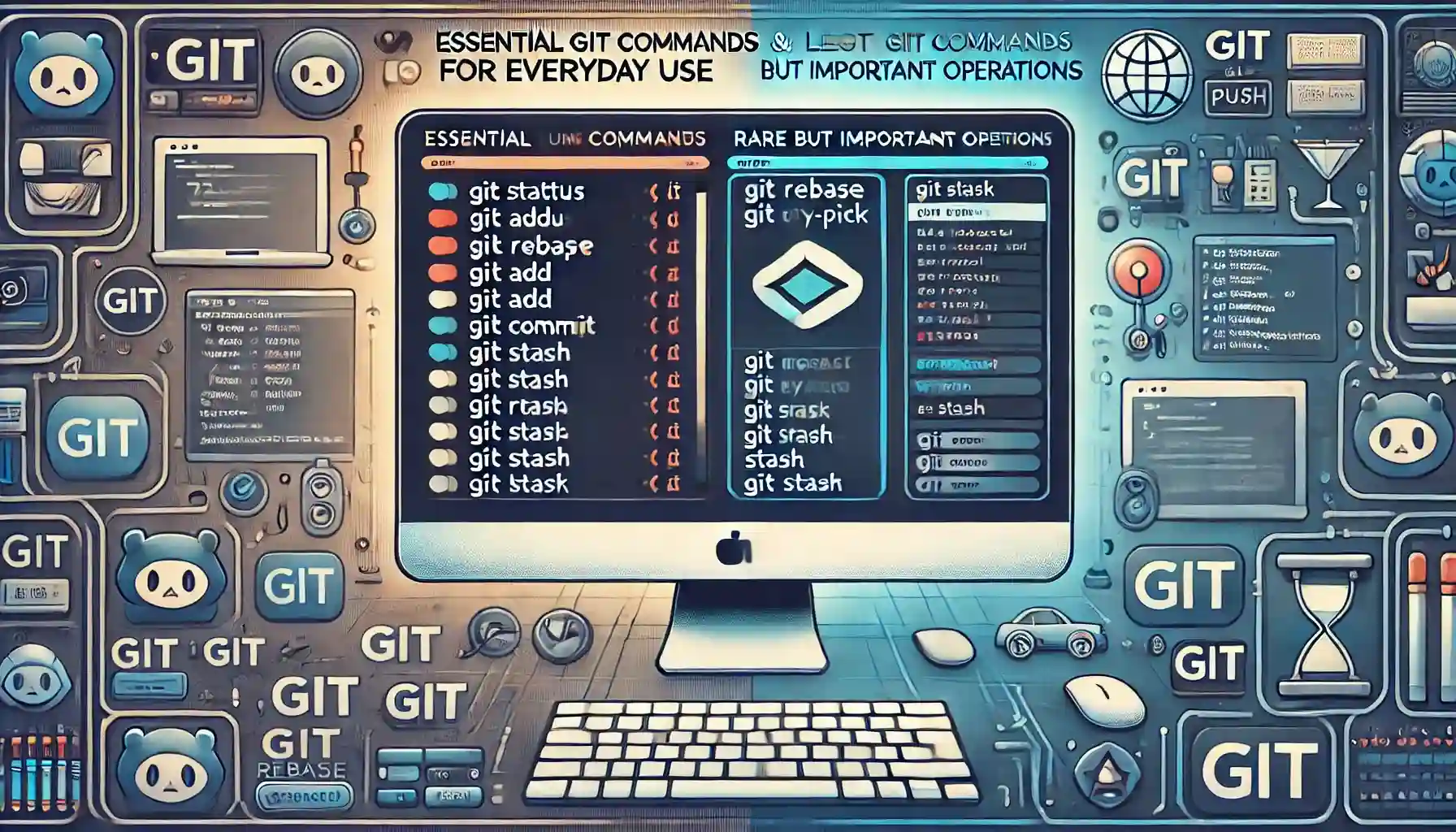
main: A---B---C---D---E
\ /
feature: F---G---H
Navigating and Managing Directories with Command Line
-
Prints the current working directory, showing the full path of the directory you are in:
pwd
-
Changes directory:
cd
-
Changes the current directory to the previous directory you were in:
cd -
-
Navigate to the parent directory:
Moves up one level in the directory structure to the parent directory.cd ../
-
List directory contents:
ls ~/desktop
-
Open the current directory in File Explorer:
start .
-
Navigate to a directory with autocompletion:
cd ~/dir + TAB
Git configuration
Configure your Git settings to personalize your commits. Proper configuration ensures that your identity is attached to your work, which is crucial for collaboration, accountability, and project management. By setting a global username and email, you make sure that all your commits across different repositories are correctly attributed to you.
-
Write the name to config:
Sets the global username for all git repositories on the system.git config --global user.name "name"
-
Write email to config:
Sets the global email for all git repositories on the system.git config --global user.email "yourEmail"
-
Check the config data:
Lists all the configuration settings for git.git config --list
-
View Git User Configuration:
command displays the contents of the Git configuration file, which contains user data and settingsCat ~/.gitconfig
Working with SSH Keys for GitHub
This block provides a step-by-step guide on how to generate, add, and test SSH keys for secure authentication with GitHub. Follow these commands to set up and verify your SSH keys.
-
create folder for SSH:
Check if .ssh folder alredy existcd ~/.ssh ls
If we don’t have this folder or file inside - createmkdir ~/.ssh
-
Generate a new SSH key:
Creates a new SSH key for secure authentication.ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
Text key name, usually id_rsa, enter the password if need. -
Add SSH key to the ssh-agent:
Starts the ssh-agent in the background and adds your SSH private key to it.eval "$(ssh-agent -s)" ssh-add ~/.ssh/id_rsa
-
Add SSH key to your GitHub account:
Go to GitHub > Settings > SSH and GPG keys, and paste your SSH public key. For copy your public key:Cat keyname.pub
-
Test SSH connection:
erifies that your SSH key is correctly added and working by testing the connection to GitHub.ssh -T git@github.com
-
Test SSH connection with a specific key:
ssh -T -i ~/.ssh/keyname git@github.com
Basic Git Commands
Working with Repositories
-
Initialize a new repository:
git init
-
Clone an existing repository:
git clone https://github.com/user/repo.git
Managing Files
-
Add a file to staging area:
git add filename
-
Add all files to staging area:
git add .
-
Commit changes with a message:
git commit -m "Commit message"
-
Commit all changes with a message:
git commit -a -m "Commit message"
Branching and Merging
-
Create a new branch:
git branch branch_name
-
Switch to another branch:
git checkout branch_name
-
Create and switch to a new branch:
git checkout -b branch_name
-
Merge a branch into the current branch:
git merge branch_name
-
Delete a branch:
git branch -d branch_name
Working with Remote Repositories
-
Show remote repositories:
git remote -v
-
Add a remote repository:
git remote add origin https://github.com/user/repo.git
-
Fetch changes from a remote repository:
git fetch origin
-
Pull changes from a remote repository into the current branch:
git pull origin branch_name
-
Push changes to a remote repository:
git push origin branch_name
Viewing History
-
DShow commit history:
git log
-
Show commit history with a graph:
git log --graph --oneline --all
Undoing Changes
-
Discard changes in the working directory:
git checkout -- filename
-
Remove the last commit but keep changes in the working directory:
git reset --soft HEAD~1
-
emove the last commit and discard changes:
git reset --hard HEAD~1
-
Reset changes in a specific file:
git checkout HEAD -- filename